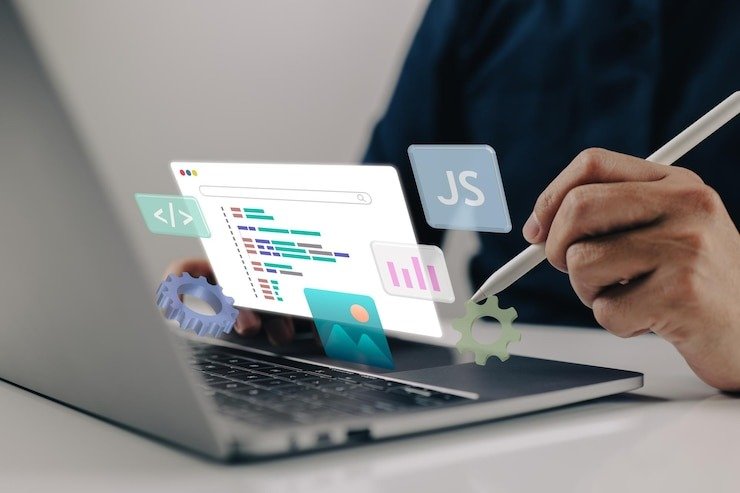
Webpack Build: How to Exclude Test Files and Optimize Your Bundles
Webpack Build: How to Exclude Test Files and Optimize Your Bundles
In modern web development, efficient builds are crucial for performance and maintainability. When working with tools like Webpack, managing which files are included in your build process can significantly affect the final bundle size and the performance of your application. This article delves into the essential practices of excluding test files and unnecessary modules from your Webpack build, comparing Webpack with Esbuild, and exploring strategies for reducing your build size.
Understanding Chromebook Partitioning: Is It Necessary and How to Do It?
How to Download PCSX2 on Chromebook: A Step-by-Step Guide
Understanding webpack and Esbuild
Webpack is a powerful module bundler for JavaScript applications. It takes modules with dependencies and generates static assets representing those modules. Webpack is highly configurable and allows developers to control almost every aspect of the build process. This flexibility, however, can make it complex for beginners.
Esbuild, on the other hand, is a relatively new bundler that focuses on speed. Written in Go, Esbuild is designed to be extremely fast, often outperforming Webpack in build times. Despite being more rapid, Esbuild is less flexible than Webpack but is an excellent choice for projects requiring quick builds with minimal configuration.
Critical Differences Between Webpack and Esbuild
- Speed: Esbuild is significantly faster than Webpack due to its Go-based architecture.
- Flexibility: Webpack offers more options and configurations, making it suitable for complex projects.
- Community Support: Webpack has a larger community and more plugins available.
- Learning Curve: Webpack can be more challenging to master due to its extensive configuration options.
Both tools are excellent for different scenarios, and the choice between Webpack and Esbuild largely depends on the specific needs of your project.
How to Exclude Test Files from Your Webpack Build
Including test files in your production build can lead to larger bundle sizes, negatively impacting your application’s performance. Fortunately, Webpack provides several methods to exclude these files.
Excluding Test Files Using ignorePatterns
One of the most straightforward ways to exclude test files is using the ignorePatterns option in your webpack configuration. You can specify patterns to match the files you want to exclude. For Example:
javascript
module.exports = {
// Other configuration options…
module: {
rules: [
{
test: /\.js$/,
exclude: [/node_modules/, /.*\.test\.js$/],
use: {
loader: ‘babel-loader’,
},
},
],
},
};
Any file that matches *.test.js will be excluded from the build process in this configuration. This is useful if you follow a naming convention for your test files.
Excluding Test Files Using externals
Another method to exclude files from your bundle is to mark them as external. When a module is marked as external, Webpack does not include it in the bundle but expects it to be available in the environment at runtime.
javascript
module.exports = {
// Other configuration options…
externals: {
‘some-test-library’: ‘commonjs some-test-library’,
},
};
This approach is beneficial for excluding entire libraries or modules that are only used in testing and not required in production.
Webpack Plugins for Excluding Files
Webpack’s rich ecosystem includes plugins to help you manage file inclusion and exclusion more effectively. One such plugin is the webpack-node-externals plugin, which automatically excludes node_modules from your bundle.
javascript
const nodeExternals = require(‘webpack-node-externals’);
module.exports = {
// Other configuration options…
externals: [nodeExternals()],
};
This configuration ensures that all files in node_modules are excluded from the build, reducing the bundle size and improving build times.
Should Webpack Ignore node_modules?
A common question among developers is whether Webpack should ignore the node_modules directory during the build process. The answer is yes, but there are some caveats.
Benefits of Excluding node_modules
- Performance: Excluding node_modules can significantly speed up the build process, as it prevents Webpack from processing many files that are usually unnecessary in the final bundle.
- Bundle Size: The files in node_modules are often large and unoptimized, so including them in your bundle can lead to unnecessarily large files.
Potential Issues with Excluding node_modules
- Missing Dependencies: If you exclude node_modules without careful consideration, you might have missing dependencies in your final build, leading to runtime errors.
- Tree Shaking: Webpack’s tree-shaking capabilities can sometimes eliminate unused code in node_modules, which you would lose out on if you excluded the entire directory.
The Right Approach
The best practice is to exclude node_modules and ensure that essential dependencies are correctly included in your bundle. This can be done by carefully configuring your Webpack rules and using plugins like webpack-node-externals to manage the exclusion intelligently.
How to Make Your Webpack Build Smaller
Reducing the size of your Webpack build is crucial for improving load times and overall application performance. Several strategies can help you achieve this.
Tree Shaking
Tree shaking is a technique that removes unused code from your final bundle. Webpack performs tree shaking by default, but it’s essential to ensure that your code is structured in a way that allows Webpack to eliminate dead code effectively.
javascript
//Example of a module that can be tree-shaken
export const used function = () => {
//Function logic
};
export const unused function = () => {
// This Function will be removed if not used
};
By using ES6 modules and ensuring that only the necessary parts of your libraries are imported, you can help Webpack shake off unused code.
Code Splitting
Code splitting is another powerful feature of Webpack that allows you to split your code into smaller chunks, which can be loaded on demand. This reduces the initial load time of your application.
javascript
// Example of dynamic import for code splitting
import(/* webpackChunkName: “my-chunk-name” */ ‘./my-module’).then(module => {
// Use the module
});
With code splitting, you can load only the code needed for the current view, keeping the initial bundle size small.
Compression Plugins
Using plugins like compression-webpack-plugin, you can compress your assets to reduce the bundle size further.
javascript
const CompressionPlugin = require(‘compression-webpack-plugin’);
module.exports = {
// Other configuration options…
plugins: [
new CompressionPlugin({
test: /\.js(\?.*)?$/i,
}),
],
};
This plugin compresses your JavaScript files using gzip or Brotli, reducing the size of the files that the client needs to download.
Excluding Modules and Files in Webpack and Esbuild
Webpack and Esbuild offer ways to exclude files or entire modules from your build. This is especially useful when you have files not needed in production or want to prevent significant dependencies from being bundled.
Webpack Exclude Options
In Webpack, you can exclude files using the exclude property in your module rules:
javascript
module.exports = {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: ‘babel-loader,’
},
],
},
};
Additionally, as mentioned earlier, you can use the external property to exclude entire modules.
Esbuild Ignore Import
In Esbuild, you can achieve similar results by marking paths as external. This prevents Esbuild from including them in the final bundle.
javascript
build.build({
entry points: [‘src/index.js’],
bundle: true,
external: [‘dash],
out file: ‘dist/bundle.js,’
}).catch(() => process.exit(1));
In this Example, Lodash is marked as external and will not be included in the final bundle. Instead, Esbuild expects it to be available at runtime.
Tsup Exclude Test Files
Tsup is another bundler that simplifies the build process. To exclude test files in Tsup, you can use the –ignore option:
bash
tsup src/index.ts –ignore ‘**/*.test.ts’
This command excludes all files that match the pattern **/*.test.ts from the build, similar to Webpack’s ignorePatterns.
Best Practices for Managing Exclusions in Webpack and Esbuild
Following best practices when managing exclusions in Webpack and Esbuild is essential to ensuring your build remains efficient and error-free.
Use Specific Patterns
Be as specific as possible when excluding files. Instead of excluding directories like src/tests, target only the files matching a particular pattern, such as *.test.js. This ensures that you don’t accidentally exclude files necessary for your build.
Test Your Build
Always test your build after making changes to exclusion rules. This helps you catch any errors that arise from missing dependencies or files excluded incorrectly.
Optimize Dependency Management
Regularly audit your dependencies to ensure you do not include unnecessary packages in your build. Tools like webpack-bundle-analyzer can help you visualize your bundle and identify significant or unused dependencies.
Questions & Answers
Q1: What is the best way to exclude test files in Webpack?
The best way to exclude test files in Webpack is to use the exclude option in your module rules. You can also use the externals property or plugins like webpack-node-externals for more control.
Q2: Should I always exclude node_modules from my Webpack build?
Excluding node_modules can improve build performance and reduce bundle size, but you should ensure that essential dependencies are included in your bundle to avoid runtime errors.
Q3: How does Esbuild compare to Webpack regarding speed and flexibility?
Esbuild is faster than Webpack but less flexible. Webpack offers more configuration options and a larger ecosystem of plugins, making it more suitable for complex projects.
Q4: What are some common mistakes to avoid when excluding files in Webpack?
Common mistakes include excluding necessary dependencies or entire directories without checking for essential files. Always test your build after making exclusions to catch potential issues.
Final Thoughts
Optimizing your Webpack build by excluding test files, unnecessary modules, and external dependencies is crucial for maintaining a lean and efficient application. While Webpack offers extensive configurability, tools like Esbuild and Tsup provide more straightforward and faster alternatives, depending on your project’s needs.
Understanding when and how to exclude specific files from your build can significantly improve build time and application performance. By following best practices and using the right tools, you can ensure that your builds are optimized and maintainable.